资料介绍
描述
在这个项目中,我们将使用适用于 Windows 10 IoT Core on Raspberry Pi 2套件组件的 Adafruit 入门包来创建一个使用气压传感器读取温度、压力和高度的项目。
注意:该套件有两个版本,一个包含 BMP280 传感器,另一个包含 BME280。如果你有 BME280,
硬件
根据下面“原理图”部分中的 Fritzing 图,将 Raspberry Pi2 连接到面包板和其他组件。
软件
您可以从https://github.com/ms-iot/adafruitsample下载代码启动项目,我们将引导您完成添加与 Web 服务对话并在地图上获取您的 pin 所需的代码。什么地图?
打开“Lesson_203\StartSolution\lesson_203.sln ”并打开 mainpage.xaml.cs 文件。
我们已经填写了一些方法作为您在此解决方案中的起点。如果你想跳到前面,你可以在以下位置找到所有代码已完成的解决方案:“Lesson_203\FullSolution\lesson_203.sln”
MainPage.xaml.cs
打开 MainPage.xaml.cs 文件。
添加对气压传感器 (BMP280) 类的引用。
public sealed partial class MainPage : Page
{
//A class which wraps the barometric sensor
BMP280 BMP280;
现在我们在 OnNavigatedTo 方法中添加代码,该方法将为气压传感器创建一个新的 BMP280 对象并初始化该对象。
如果您不想在地图上添加图钉,请删除 MakePinWebAPICall();
//This method will be called by the application framework when the page is first loaded
protected override async void OnNavigatedTo(NavigationEventArgs navArgs)
{
Debug.WriteLine("MainPage::OnNavigatedTo");
MakePinWebAPICall();
try
{
//Create a new object for our barometric sensor class BMP280 = new BMP280();
//Initialize the sensor
await BMP280.Initialize();
接下来我们添加代码来执行以下操作:
- 创建变量来存储温度、压力和高度。将它们设置为 0。
- 为海平面压力创建一个变量。默认值为 1013.25 hPa。
- 读取温度、压力和高度 10 次并将值输出到调试控制台。
//Create variables to store the sensor data: temperature, pressure and altitude.
//Initialize them to 0.
float temp = 0;
float pressure = 0;
float altitude = 0;
//Create a constant for pressure at sea level.
//This is based on your local sea level pressure (Unit: Hectopascal)
const float seaLevelPressure = 1013.25f;
//Read 10 samples of the data
for(int i = 0; i < 10; i++)
{
temp = await BMP280.ReadTemperature();
pressure = await BMP280.ReadPreasure();
altitude = await BMP280.ReadAltitude(seaLevelPressure);
//Write the values to your debug console
Debug.WriteLine("Temperature: " + temp.ToString() + " deg C");
Debug.WriteLine("Pressure: " + pressure.ToString() + " Pa");
Debug.WriteLine("Altitude: " + altitude.ToString() + " m");
}
}
catch (Exception ex)
{
Debug.WriteLine(ex.Message);
}
BMP280.cs
打开 BMP280.cs 文件。
代码的第一部分是列出 BMP280 中不同寄存器的地址。这些值可以在BMP280 数据表中找到。
在 BMP280 类中,在寄存器地址枚举之后添加以下内容。
//String for the friendly name of the I2C bus
const string I2CControllerName = "I2C1";
//Create an I2C device
private I2cDevice bmp280 = null;
//Create new calibration data for the sensor
BMP280_CalibrationData CalibrationData;
//Variable to check if device is initialized
bool init = false;
接下来在Initialize函数中添加如下代码:
//Method to initialize the BMP280 sensor
public async Task Initialize()
{
Debug.WriteLine("BMP280::Initialize");
try
{
//Instantiate the I2CConnectionSettings using the device address of the BMP280
I2cConnectionSettings settings = new I2cConnectionSettings(BMP280_Address);
//Set the I2C bus speed of connection to fast mode settings.BusSpeed = I2cBusSpeed.FastMode;
//Use the I2CBus device selector to create an advanced query syntax string
string aqs = I2cDevice.GetDeviceSelector(I2CControllerName); //Use the Windows.Devices.Enumeration.DeviceInformation class to create a collection using the advanced query syntax string
DeviceInformationCollection dis = await DeviceInformation.FindAllAsync(aqs);
//Instantiate the the BMP280 I2C device using the device id of the I2CBus and the I2CConnectionSettings
bmp280 = await I2cDevice.FromIdAsync(dis[0].Id, settings);
//Check if device was found
if (bmp280 == null)
{
Debug.WriteLine("Device not found");
}
}
catch (Exception e)
{
Debug.WriteLine("Exception: " + e.Message + "\n" + e.StackTrace);
throw;
}
}
在Begin函数中添加如下代码:
private async Task Begin()
{
Debug.WriteLine("BMP280::Begin");
byte[] WriteBuffer = new byte[] { (byte)eRegisters.BMP280_REGISTER_CHIPID };
byte[] ReadBuffer = new byte[] { 0xFF };
//Read the device signature
bmp280.WriteRead(WriteBuffer, ReadBuffer);
Debug.WriteLine("BMP280 Signature: " + ReadBuffer[0].ToString()); //Verify the device signature
if (ReadBuffer[0] != BMP280_Signature)
{
Debug.WriteLine("BMP280::Begin Signature Mismatch.");
return;
}
//Set the initalize variable to true
init = true;
//Read the coefficients table
CalibrationData = await ReadCoefficeints();
//Write control register
await WriteControlRegister();
//Write humidity control register
await WriteControlRegisterHumidity();
}
将以下代码添加到接下来的 2 个函数中以写入控制寄存器。
//Method to write 0x03 to the humidity control register
private async Task WriteControlRegisterHumidity()
{
byte[] WriteBuffer = new byte[] { (byte)eRegisters.BMP280_REGISTER_CONTROLHUMID, 0x03 };
bmp280.Write(WriteBuffer);
await Task.Delay(1);
return;
}
//Method to write 0x3F to the control register
private async Task WriteControlRegister()
{
byte[] WriteBuffer = new byte[] { (byte)eRegisters.BMP280_REGISTER_CONTROL, 0x3F };
bmp280.Write(WriteBuffer);
await Task.Delay(1);
return;
}
将以下代码添加到 ReadUInt16_LittleEndian 函数中即可:
//Method to read a 16-bit value from a register and return it in little endian format
private UInt16 ReadUInt16_LittleEndian(byte register)
{
UInt16 value = 0;
byte[] writeBuffer = new byte[] { 0x00 };
byte[] readBuffer = new byte[] { 0x00, 0x00 };
writeBuffer[0] = register;
bmp280.WriteRead(writeBuffer, readBuffer);
int h = readBuffer[1] << 8;
int l = readBuffer[0];
value = (UInt16)(h + l);
return value;
}
将以下代码添加到 ReadByte 函数以从寄存器中读取 8 位数据。
//Method to read an 8-bit value from a register
private byte ReadByte(byte register)
{
byte value = 0;
byte[] writeBuffer = new byte[] { 0x00 };
byte[] readBuffer = new byte[] { 0x00 };
writeBuffer[0] = register;
bmp280.WriteRead(writeBuffer, readBuffer);
value = readBuffer[0];
return value;
}
接下来的 3 个功能已为您完成。可以在数据表中找到编写这些函数所需的信息。
- ReadCoefficeints:这是从寄存器地址读取所有校准数据的函数。
- BMP280_compensate_T_double:在此函数中,使用 BMP280 数据表中的补偿公式计算以 ºC 为单位的温度。
- BMP280_compensate_P_Int64:在此函数中,使用 BMP280 数据表中的补偿公式计算以 Pa 为单位的压力。
添加如下代码完成ReadTemperature功能。
public async Task<float> ReadTemperature()
{
//Make sure the I2C device is initialized
if (!init) await Begin();
//Read the MSB, LSB and bits 7:4 (XLSB) of the temperature from the BMP280 registers
byte tmsb = ReadByte((byte)eRegisters.BMP280_REGISTER_TEMPDATA_MSB);
byte tlsb = ReadByte((byte)eRegisters.BMP280_REGISTER_TEMPDATA_LSB);
byte txlsb = ReadByte((byte)eRegisters.BMP280_REGISTER_TEMPDATA_XLSB); // bits 7:4
//Combine the values into a 32-bit integer
Int32 t = (tmsb << 12) + (tlsb << 4) + (txlsb >> 4);
//Convert the raw value to the temperature in degC
double temp = BMP280_compensate_T_double(t);
//Return the temperature as a float value
return (float)temp;
}
重复相同的步骤以完成 ReadPressure 函数。
public async Task<float> ReadPreasure()
{
//Make sure the I2C device is initialized
if (!init) await Begin();
//Read the temperature first to load the t_fine value for compensation
if (t_fine == Int32.MinValue)
{
await ReadTemperature();
}
//Read the MSB, LSB and bits 7:4 (XLSB) of the pressure from the BMP280 registers
byte tmsb = ReadByte((byte)eRegisters.BMP280_REGISTER_PRESSUREDATA_MSB);
byte tlsb = ReadByte((byte)eRegisters.BMP280_REGISTER_PRESSUREDATA_LSB);
byte txlsb = ReadByte((byte)eRegisters.BMP280_REGISTER_PRESSUREDATA_XLSB); // bits 7:4
//Combine the values into a 32-bit integer
Int32 t = (tmsb << 12) + (tlsb << 4) + (txlsb >> 4);
//Convert the raw value to the pressure in Pa
Int64 pres = BMP280_compensate_P_Int64(t);
//Return the temperature as a float value
return ((float)pres) / 256;
}
最后完成ReadAltitude函数:
//Method to take the sea level pressure in Hectopascals(hPa) as a parameter and calculate the altitude using current pressure.
public async Task<float> ReadAltitude(float seaLevel)
{
//Make sure the I2C device is initialized
if (!init) await Begin();
//Read the pressure first
float pressure = await ReadPreasure();
//Convert the pressure to Hectopascals(hPa)
pressure /= 100;
//Calculate and return the altitude using the international barometric formula
return 44330.0f * (1.0f - (float)Math.Pow((pressure / seaLevel), 0.1903f));
}
您的项目现在可以部署了!
预期产出
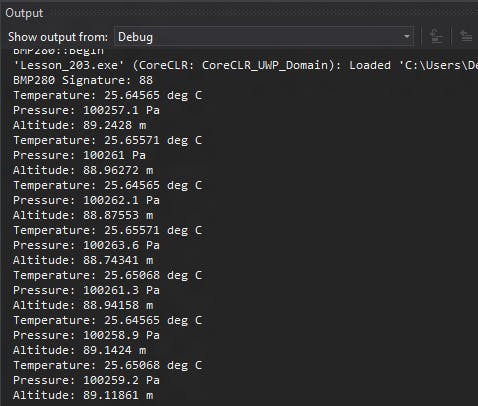
在这里查看下一课。
- 气压传感器BMP数据手册下载 8次下载
- 高精度低功耗的小型双气压计芯片SPL06-007 0次下载
- P900系列高性能金属应变式压力传感器的中文数据手册免费下载 15次下载
- 进气压力传感器试验箱说明 5次下载
- 集成压力传感器的无源胎压监控 13次下载
- FM-DQY大气压力传感器说明 8次下载
- P900系列高性能金属应变式压力传感器 12次下载
- 光纤压力和温度传感器在石油天然气应用 36次下载
- 如何实现压阻式压力传感器温度的补偿方法 42次下载
- BMP085气压传感器的详细资料介绍和应用代码免费下载 23次下载
- 进气压力传感器 24次下载
- 用硅压阻式压力传感器解算高度速度的方法 24次下载
- 气压和流速对微热板气体传感器的影响 12次下载
- 轮胎气压传感器设计 87次下载
- 用双电桥法减小压力传感器输出的温度漂移 34次下载
- HPX气体压力传感器的功能特性及如何实现飞行器高度测量 3869次阅读
- 单总线数字温度传感器DS18B20时序的温度采集与读取研究 4368次阅读
- 进气压力传感器输出特性_进气压力传感器原理 3029次阅读
- 进气压力传感器坏了有哪些反应 1w次阅读
- 进气压力传感器的作用及安装位置 2.1w次阅读
- 进气压力传感器信号电路电压过高的原因 3.4w次阅读
- 进气压力传感器怎么测量好坏 1.9w次阅读
- 进气压力传感器故障现象及解决方法 2w次阅读
- 气压传感器的应用领域_气压传感器的作用 3850次阅读
- 气压传感器的工作原理_气压传感器应用 2.3w次阅读
- 进气压力传感器坏了有什么反应 2.1w次阅读
- 温度传感器和压力传感器在智能啤酒桶中的应用 3609次阅读
- VTI公司的压力传感器让飞行员手表实现了精确气压和高度测量的功能 1233次阅读
- MEMS气压传感器的工作原理及在物联网中的应用 5746次阅读
- 高度传感器的应用_高度传感器的作用 1.9w次阅读
下载排行
本周
- 1山景DSP芯片AP8248A2数据手册
- 1.06 MB | 532次下载 | 免费
- 2RK3399完整板原理图(支持平板,盒子VR)
- 3.28 MB | 339次下载 | 免费
- 3TC358743XBG评估板参考手册
- 1.36 MB | 330次下载 | 免费
- 4DFM软件使用教程
- 0.84 MB | 295次下载 | 免费
- 5元宇宙深度解析—未来的未来-风口还是泡沫
- 6.40 MB | 227次下载 | 免费
- 6迪文DGUS开发指南
- 31.67 MB | 194次下载 | 免费
- 7元宇宙底层硬件系列报告
- 13.42 MB | 182次下载 | 免费
- 8FP5207XR-G1中文应用手册
- 1.09 MB | 178次下载 | 免费
本月
- 1OrCAD10.5下载OrCAD10.5中文版软件
- 0.00 MB | 234315次下载 | 免费
- 2555集成电路应用800例(新编版)
- 0.00 MB | 33566次下载 | 免费
- 3接口电路图大全
- 未知 | 30323次下载 | 免费
- 4开关电源设计实例指南
- 未知 | 21549次下载 | 免费
- 5电气工程师手册免费下载(新编第二版pdf电子书)
- 0.00 MB | 15349次下载 | 免费
- 6数字电路基础pdf(下载)
- 未知 | 13750次下载 | 免费
- 7电子制作实例集锦 下载
- 未知 | 8113次下载 | 免费
- 8《LED驱动电路设计》 温德尔著
- 0.00 MB | 6656次下载 | 免费
总榜
- 1matlab软件下载入口
- 未知 | 935054次下载 | 免费
- 2protel99se软件下载(可英文版转中文版)
- 78.1 MB | 537798次下载 | 免费
- 3MATLAB 7.1 下载 (含软件介绍)
- 未知 | 420027次下载 | 免费
- 4OrCAD10.5下载OrCAD10.5中文版软件
- 0.00 MB | 234315次下载 | 免费
- 5Altium DXP2002下载入口
- 未知 | 233046次下载 | 免费
- 6电路仿真软件multisim 10.0免费下载
- 340992 | 191187次下载 | 免费
- 7十天学会AVR单片机与C语言视频教程 下载
- 158M | 183279次下载 | 免费
- 8proe5.0野火版下载(中文版免费下载)
- 未知 | 138040次下载 | 免费
评论