1.map/multimap容器简介
map/multimap容器,也是一个关联式容器,底层通过二叉树实现。
map/multimap容器中保存的是对组(pair)数据。对组(pair)的第一个元素键值key,起到索引作用,第二个元素value为实值;
map容器第一个键值不能重复,出现重复则会自动忽略该数据,multimap则不受此限制;
map/multimap插入数据时会根据键值key进行排序;
2.map/multimap容器构造函数与赋值
-
map/multimap构造函数:
map p;无参构造
map(begin,end);//区间赋值
map(const map &p);//拷贝构造,type> -
map/multimap赋值:
重载"=": map &operator=(); -
map/multimap插入数据:
inset()
使用示例:
#include < iostream >
#include < map >
using namespace std;
#if 0
void Printmap(map< int, string >& p)
{
for (map< int, string >::iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout < < "key=" < < ptr- >first < < "tvalue=" < < (*ptr).second < < endl;
}
}
void test()
{
map< int, string >p1;
p1.insert(pair< int, string >(1, "小王"));
p1.insert(pair< int, string >(3,"小李"));
p1.insert(pair< int, string >(2, "小刘"));
p1.insert(pair< int, string >(8, "小红"));
p1.insert(pair< int, string >(5, "小王"));
p1.insert(pair< int, string >(5, "小李"));
cout < < "无参构造,插入数据示例:" < < endl;
Printmap(p1);
map< int, string >p2(p1.begin(), p1.end());
cout < < "区间赋值p2" < < endl;
Printmap(p2);
map< int, string >p3(p1);
cout < < "拷贝构造p3" < < endl;
Printmap(p3);
}
//multimap示例:
void Printmultimap(multimap< int, string >& p)
{
for (multimap< int, string >::iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout < < "key=" < < ptr- >first < < "tvalue=" < < (*ptr).second < < endl;
}
}
void test02(void)
{
multimap< int, string >mp;
mp.insert(pair< int, string >(1, "小王"));
mp.insert(pair< int, string >(3, "小李"));
mp.insert(pair< int, string >(2, "小刘"));
mp.insert(pair< int, string >(8, "小红"));
mp.insert(pair< int, string >(5, "小王"));
mp.insert(pair< int, string >(5, "小李"));
Printmultimap(mp);
}
int main()
{
cout < < "tmap示例:" < < endl;
test();
cout < < "ntmultimap示例" < < endl;
test02();
system("pause");
}
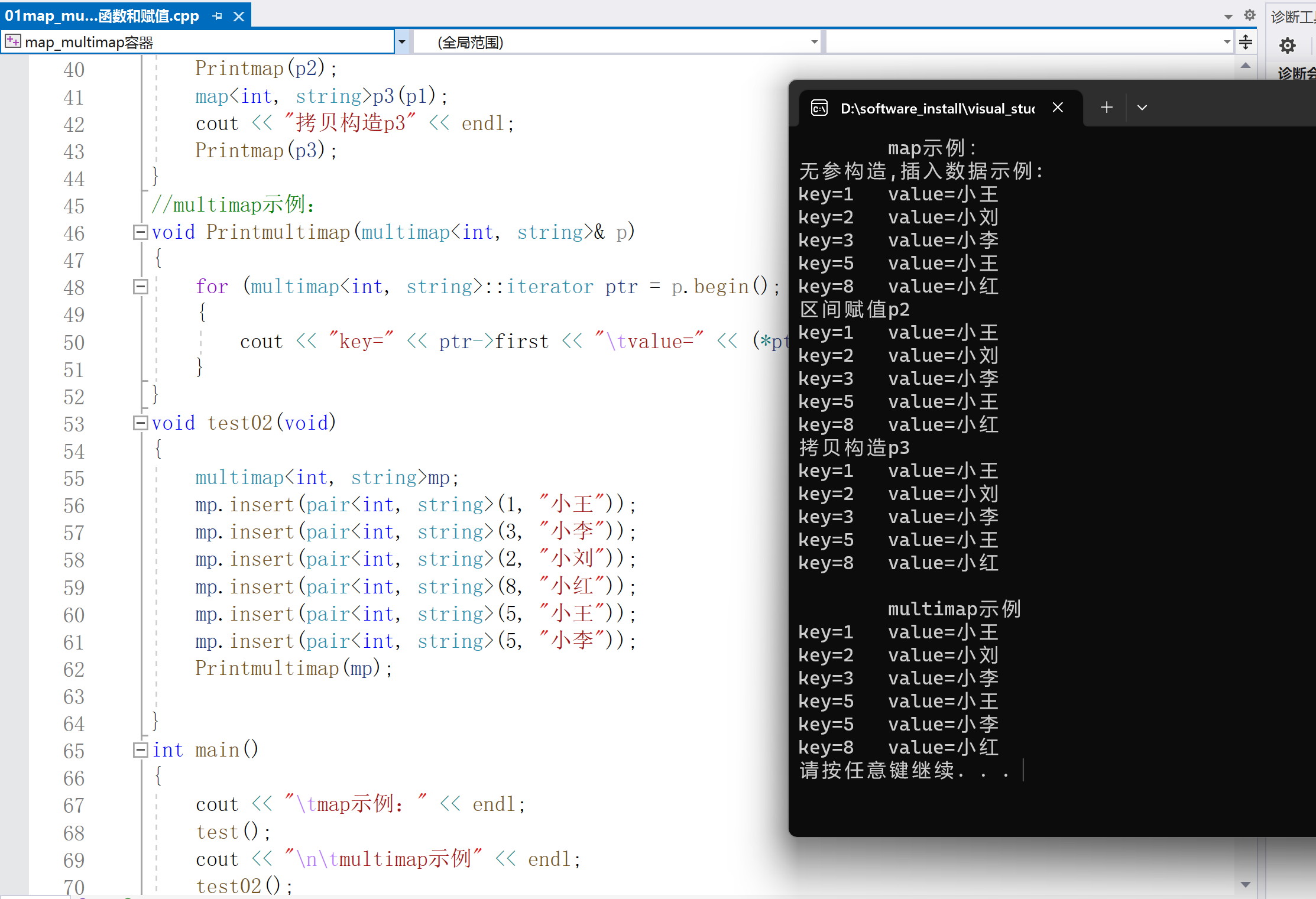
3.map/multimap获取容器元素个数和互换数据
map/multimap获取容器元素个数和互换数据:
size(); --获取元素个数
empty(); --判断容器是否为空
swap(); --互换数据
使用示例:
#include < iostream >
#include < map >
using namespace std;
void PrintMap(map< int, string >& p)
{
for (map< int, string >::iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout < < "key=" < < ptr- >first < < "tvalue=" < < ptr- >second < < endl;
}
}
void test()
{
map< int, string >p1;
p1.insert(pair< int, string >(1, "小王"));
p1.insert(pair< int, string >(3, "小李"));
p1.insert(pair< int, string >(2, "小刘"));
p1.insert(pair< int, string >(8, "小红"));
p1.insert(pair< int, string >(5, "小王"));
p1.insert(pair< int, string >(5, "小李"));
if (p1.empty())
{
cout < < "p1容器为空!" < < endl;
}
else
{
cout < < "p1容器不为空!" < < endl;
}
cout < < "p1成员个数:" < < p1.size() < < endl;
PrintMap(p1);
map< int, string >p2;
p2.insert(pair< int, string >(10, "AA"));
p2.insert(pair< int, string >(13, "BB"));
p2.insert(pair< int, string >(12, "CC"));
cout < < "p2成员个数:" < < p2.size() < < endl;
PrintMap(p1);
cout < < "互换元素" < < endl;
p1.swap(p2);
cout < < "p1成员个数:" < < p1.size() < < endl;
PrintMap(p1);
cout < < "p2成员个数:" < < p2.size() < < endl;
PrintMap(p2);
}
int main()
{
test();
system("pause");
}
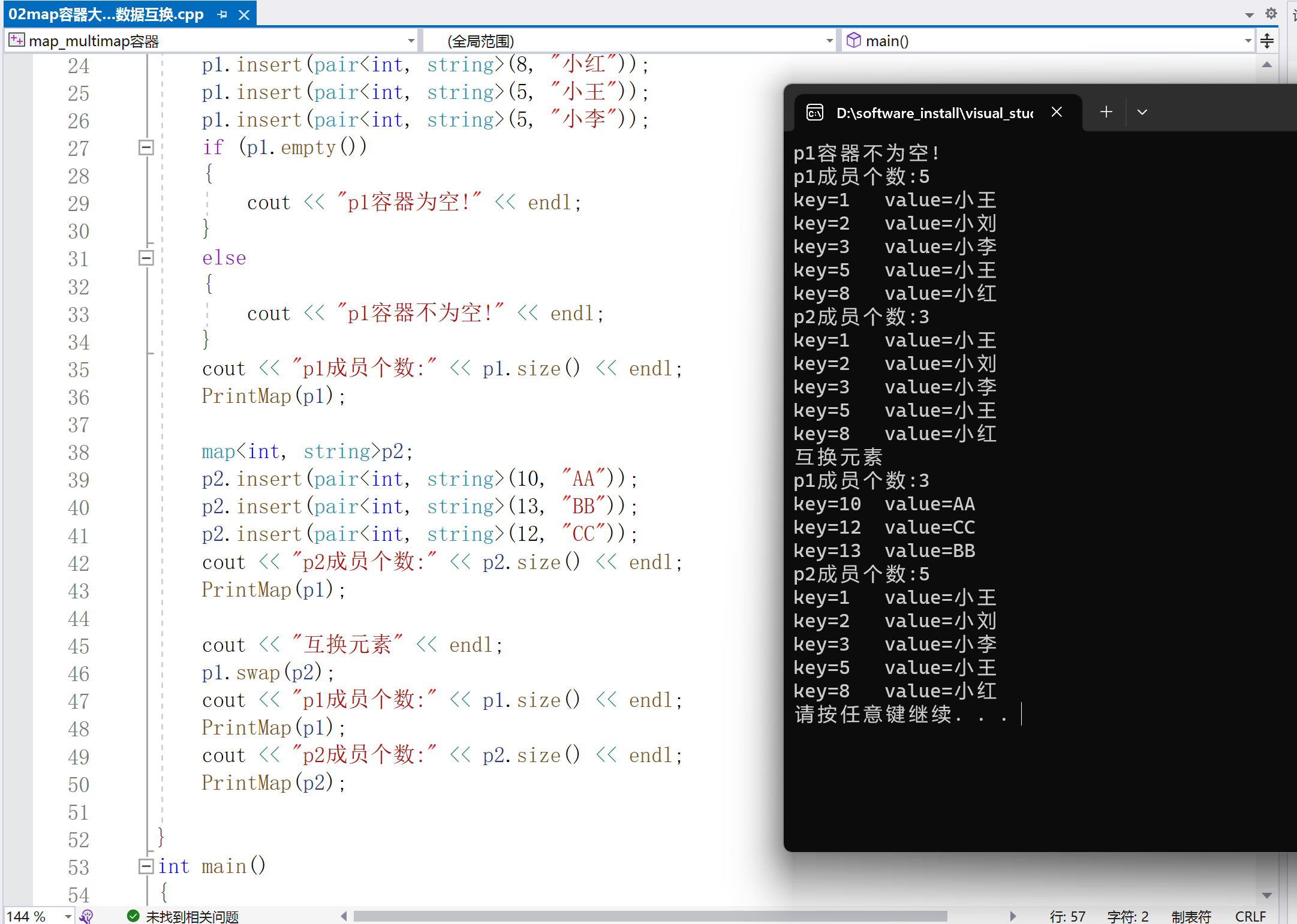
4.map/multimap插入元素和删除元素
map/multimap插入元素
insert(elem) --插入单个元素
insert(begin,end);//插入一个区间
map/multimap删除元素:
erase(pos); --删除指定位置的数据,返回下一个成员的迭代器
erase(begin,end);--删除一个区间
erase(key); --指定keyc删除
erase(elem);//删除指定元素
clear() --清空容器
使用示例:
#include < iostream >
#include < map >
using namespace std;
class Person
{
public:
Person() {
}
Person(int age, string name) :age(age), name(name) {
}
int age;
string name;
};
ostream& operator< <(ostream& cout, Person& p)
{
cout < < "姓名:" < < p.name < < "t年龄:" < < p.age < < endl;
return cout;
}
void PrintMap(const map< int, Person >& p)
{
for (map< int, Person >::const_iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout < < "key=" < < ptr- >first < < "t姓名:" < < (ptr- >second).name < < "t年龄:" < < (ptr- >second).age < < endl;
}
}
void test()
{
map< int, Person > p;
//第一种方式:
p.insert(pair< int, Person >(1, Person(18, "小王")));
//第二种方式:
p.insert(make_pair(5, Person(19, "小林")));
//第三种
p.insert(map< int, Person >::value_type(2, Person(20, "小崔")));
//第四种
p[3] = Person(20,"小刘");//一般不推荐这种方式
cout < <"p[3]内容:"< < p[3] < < endl;//可以通过[]方式访问
cout < < "p的成员信息:" < < endl;
PrintMap(p);
map< int, Person >p2;
p2.insert(p.begin(), p.end());//区间插入
cout < < "p2的成员信息:" < < endl;
PrintMap(p2);
cout < < "p2删除第2个位置的内容:" < < endl;
map< int, Person >::iterator pos = p2.begin();
for (int i = 0; i < 2; i++)pos++;
p2.erase(pos);
PrintMap(p2);
cout < < "p2删除指key值删除(key=1)" < < endl;
p2.erase(1);
PrintMap(p2);
cout < < "p2删除区间" < < endl;
p2.erase(p2.begin(),p2.end());//相当于p.clear()
PrintMap(p2);
}
int main()
{
test();
system("pause");
}
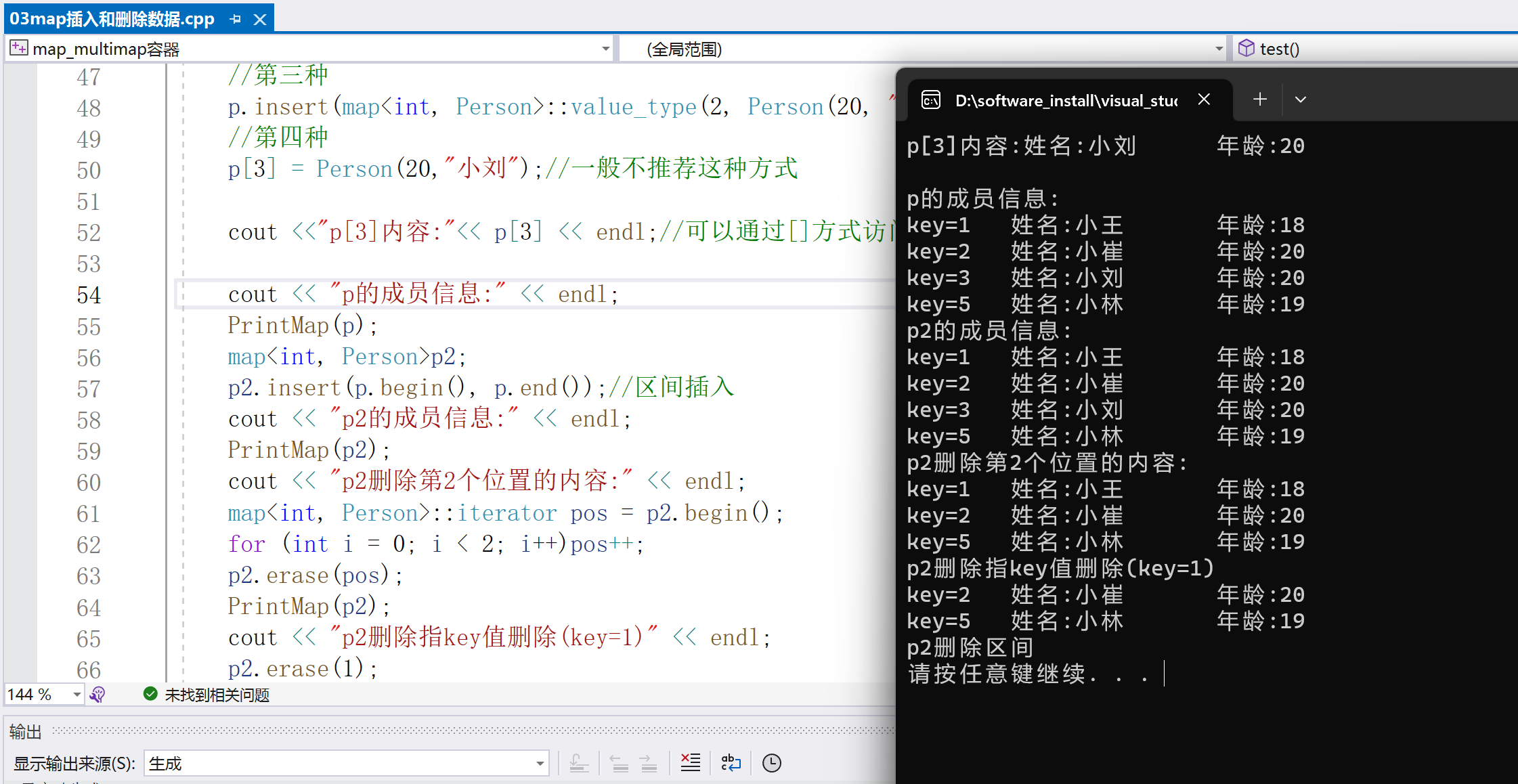
5.map/multimap查找与统计
map/multimap查找:
find(key);//按key值查找
lower_bound(key);//找到第一个大于等于key值,返回该位置的迭代器
upper_bound(key);//返回第一个大于key的值的迭代器
equal_range(key);//返回一个迭代器队组,第一个值等价于lower_bound(key)迭代器
第二个值等价于upper_bound(key)迭代器
map/multimap统计:
count(key);//按key值统计
使用示例:
#include < iostream >
using namespace std;
#include < map >
class Person
{
public:
Person() {}
Person(int age, string name) :age(age), name(name) {
}
string name;
int age;
};
ostream& operator< <(ostream& cout, Person& p)
{
cout < < "姓名:" < < p.name < < "t年龄:" < < p.age;
return cout;
}
void PrintMap(map< int, Person >& p)
{
for (map< int, Person >::iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout < < "key=" < < ptr- >first < < "t" < < ptr- >second < < endl;
}
}
void test()
{
map< int, Person >p;
p.insert(pair< int, Person >(1, Person(18, "小王")));
p.insert(make_pair(3, Person(25, "小刘")));
p.insert(map< int, Person >::value_type(2, Person(18, "小林")));
p.insert(make_pair(4, Person(20, "阿水")));
p.insert(make_pair(2, Person(20, "阿水")));
PrintMap(p);
map< int,Person >::iterator ret=p.find(2);
if (ret != p.end())
{
cout < < "查找成功!" < < endl;
cout < < "key=" < < ret- >first < < "t" < < ret- >second < < endl;
}
else
{
cout < < "未查找到!" < < endl;
}
int cnt=p.count(2);
cout < < "key=2的个数:" < < cnt < < endl;
}
void PrintmultiMap(multimap< int, Person >& p)
{
for (multimap< int, Person >::iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout < < "key=" < < ptr- >first < < "t" < < ptr- >second < < endl;
}
}
//multimap示例
void test02()
{
multimap< int, Person >mp;
mp.insert(pair< int, Person >(1, Person(18, "小王")));
mp.insert(make_pair(2, Person(25, "小刘")));
mp.insert(multimap< int, Person >::value_type(2, Person(18, "小林")));
mp.insert(make_pair(4, Person(20, "阿水")));
mp.insert(make_pair(2, Person(20, "阿水")));
cout < < "multimap成员信息" < < endl;
PrintmultiMap(mp);
multimap< int, Person >::iterator temp = mp.find(2);
cout < < "key=2的所有成员信息< 一 >:" < < endl;
for (int k = 0; k < mp.count(2); k++, temp++)
{
cout < < "key=" < < temp- >first < < "t" < < temp- >second < < endl;
}
cout < < "key=2的个数:" < < mp.count(2) < < endl;
cout < < "key=2的所有成员信息< 二 >:" < < endl;
temp = mp.lower_bound(2);
multimap< int, Person >::iterator temp2 = mp.upper_bound(2);
for (; temp != temp2; temp++)
{
cout < < "key=" < < temp- >first < < "t" < < temp- >second < < endl;
}
cout < < "key=2的所有成员信息< 三 >:" < < endl;
pair< multimap< int, Person >::iterator, multimap< int, Person >::iterator> ret2 = mp.equal_range(2);
while (ret2.first != ret2.second)
{
cout < < "key=" < < (ret2.first)- >first < < "t" < < (ret2.first)- >second < < endl;
ret2.first++;
}
}
int main()
{
cout < < "map" < < endl;
test();
cout < < "nmultimap" < < endl;
test02();
system("pause");
}
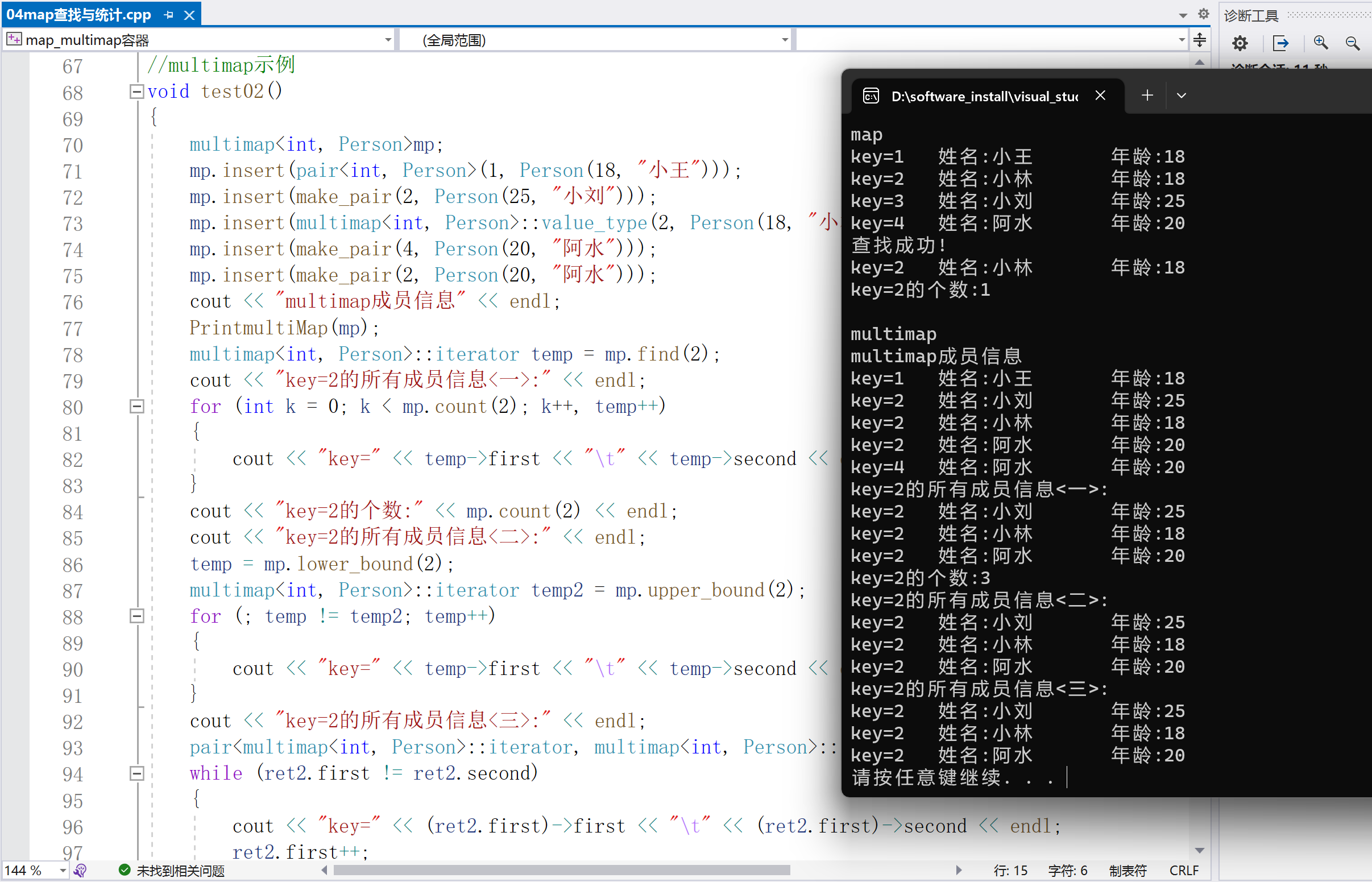
6.map/multimap指定排序
map/multimap插入数据时默认以key值排序,按照升序排序,若要改为降序排序,则需要提供一个仿函数。
#include < iostream >
using namespace std;
#include < map >
class Person
{
public:
Person() {
}
Person(int age, string name) :age(age), name(name) {
}
int age;
string name;
};
ostream& operator< <(ostream& cout, const Person& p)
{
cout < < "姓名:" < < p.name < < "t年龄:" < < p.age ;
return cout;
}
class mycompare
{
public:
bool operator()(int val1, int val2)const
{
return val1 > val2;
}
};
void PrintMap(const map< int, Person, mycompare >& p)
{
for (map< int, Person, mycompare >::const_iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout < < "key=" < < ptr- >first < < "t" < < ptr- >second < < endl;
}
}
void test01()
{
map< int, Person,mycompare >p;
p.insert(make_pair(1, Person(18, "小王")));
p.insert(make_pair(6, Person(25, "小刘")));
p.insert(make_pair(2, Person(18, "小林")));
p.insert(make_pair(4, Person(20, "阿水")));
p.insert(make_pair(3, Person(20, "阿水")));
PrintMap(p);
}
int main()
{
test01();
system("pause");
}
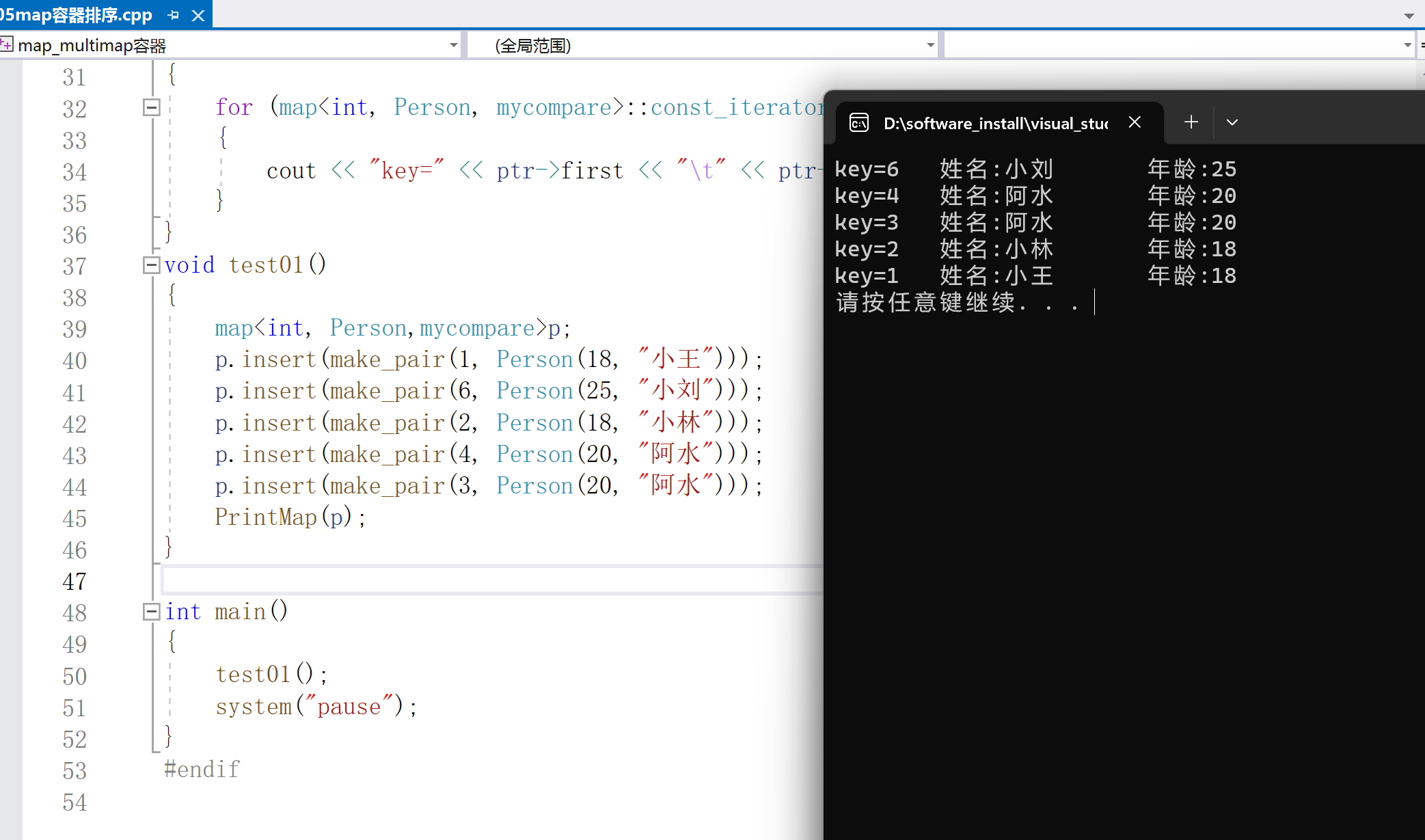
7.职工分组管理案例
公司有10个员工(ABCDEFGHIJ),现在用重新对员工进行部门调整;员工信息组成:姓名、工资,所属部门(策划、美工、研发);
1.随机给10个员工分配部门和工资;
2.通过multimap随机插入,key代表部门,value代表员工;
3.分部门显示员工信息(职工信息以工资从高到底进行排序);
- 功能实现:
(1)创建职工类,该类包含的成员有职工姓名、工资;
(2)创建一个vector容器,保存10个职工信息。姓名和工资均通过随机数生成;
(3)对vector容器进行排序,按工资进行降序排序;
(4)创建一个multimap容器,所属部门作为key,进行职工信息插入;
(5)进行分部门输出职工信息。通过lower_bound()和upper_bound()函数进行查找处理。
实现示例:
#include < iostream >
#include < map >
#include < vector >
#include < algorithm >
using namespace std;
class Worker
{
public:
Worker(string name, int money) :name(name), money(money) {
}
bool operator< (Worker p)const
{
if (this- >money == p.money)
{
return this->name < p.name;
}
return this- >money>p.money;
}
string name;//姓名
int money;//工资
};
ostream& operator< <(ostream& cout, Worker& p)
{
cout < < "姓名:" < < p.name < < "t工资:" < < p.money;
return cout;
}
//设置员工信息
void SetWorkerInfo(vector< Worker > &p)
{
char data;
int money;
for (int i = 0; i < 10; i++)
{
data = 'A' + rand() % 11;
string name;
name = data;
money = 6000 + rand() % 10000;
p.push_back(Worker(name, money));
}
//对员工按工资排序
sort(p.begin(),p.end());
}
//分配部门信息
void SetWorkerPosition(multimap< string, Worker >& p,vector< Worker >&vtr)
{
int flag = 0;
for (int i = 0; i < 10; i++)
{
flag = rand() % 3;
switch (flag)
{
case 0://策划
p.insert(make_pair("策划", vtr[i]));
break;
case 1://美工
p.insert(make_pair("美工", vtr[i]));
break;
case 2://研发
p.insert(make_pair("研发", vtr[i]));
break;
}
}
}
//分部门显示信息
void DisplayWorkerInfo(multimap< string, Worker >&p, string position)
{
multimap< string, Worker >::iterator begin = p.lower_bound(position);//找到第一个>=position内容
multimap< string, Worker >::iterator end = p.upper_bound(position);//找找到第一个>positionn内容
cout < < "t" < < position < < "部门员工 " < < p.count(position) < < " 人" < < endl;
for (; begin != end; begin++)
{
cout < < begin- >second < < endl;
}
}
int main()
{
srand(time(NULL));//生成随机数种子
vector< Worker > vtr;//创建一个vec容器
//保存员工信息
SetWorkerInfo(vtr);
/*for (vector< worker >::iterator ptr = vtr.begin(); ptr != vtr.end(); ptr++)
{
cout < < *ptr < < endl;
}*/
multimap< string, Worker >p;
SetWorkerPosition(p, vtr);
/*for (multimap< string, Worker >::iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout < < "岗位:" < < ptr- >first < < "t" < < ptr- >second < < endl;
}*/
DisplayWorkerInfo(p, "策划");
DisplayWorkerInfo(p, "美工");
DisplayWorkerInfo(p, "研发");
system("pause");
}
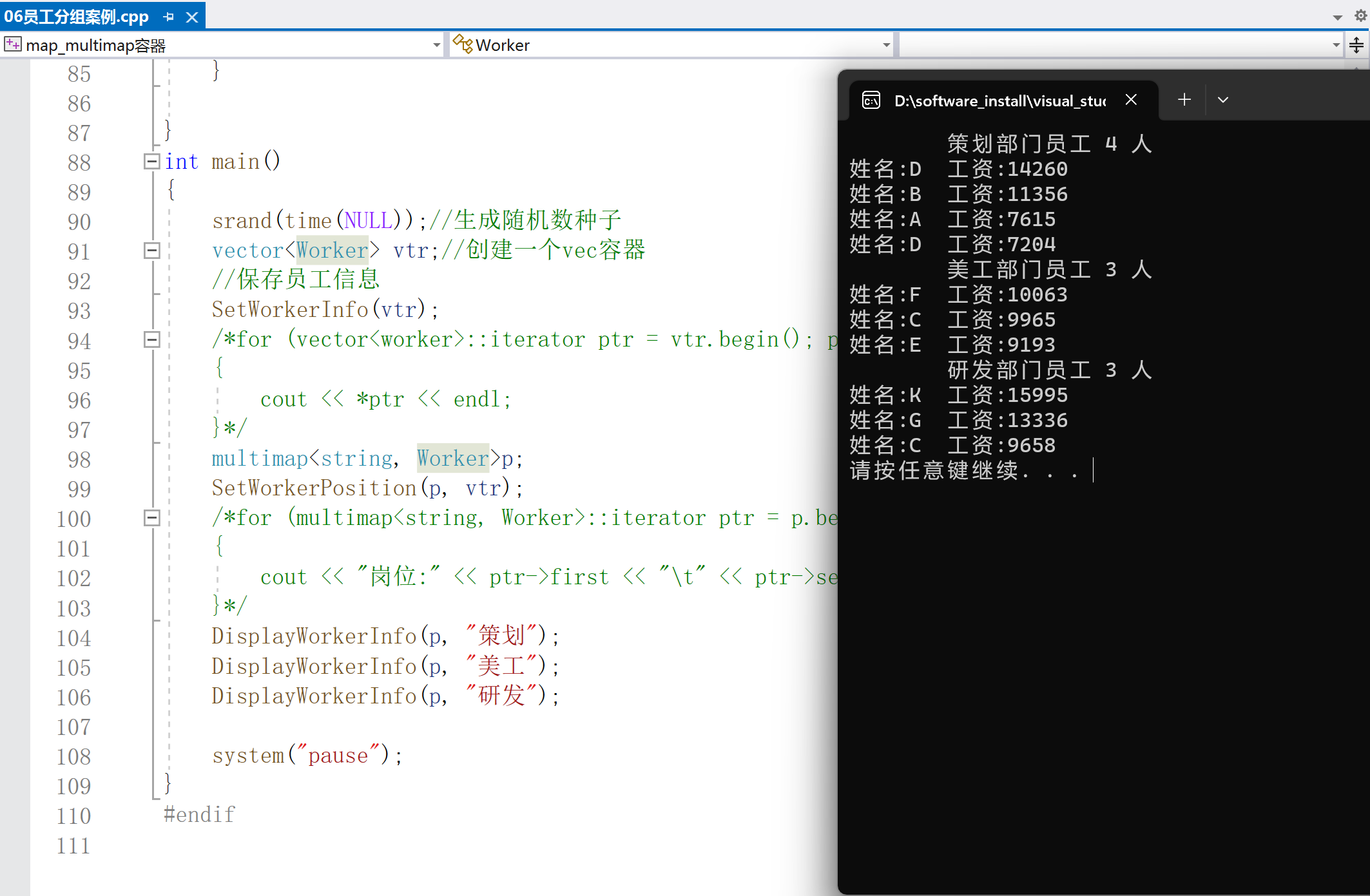
审核编辑:汤梓红
-
函数
+关注
关注
3文章
3868浏览量
61309 -
容器
+关注
关注
0文章
481浏览量
21883 -
C++
+关注
关注
21文章
2066浏览量
72900 -
MAP
+关注
关注
0文章
47浏览量
15021
发布评论请先 登录
相关推荐
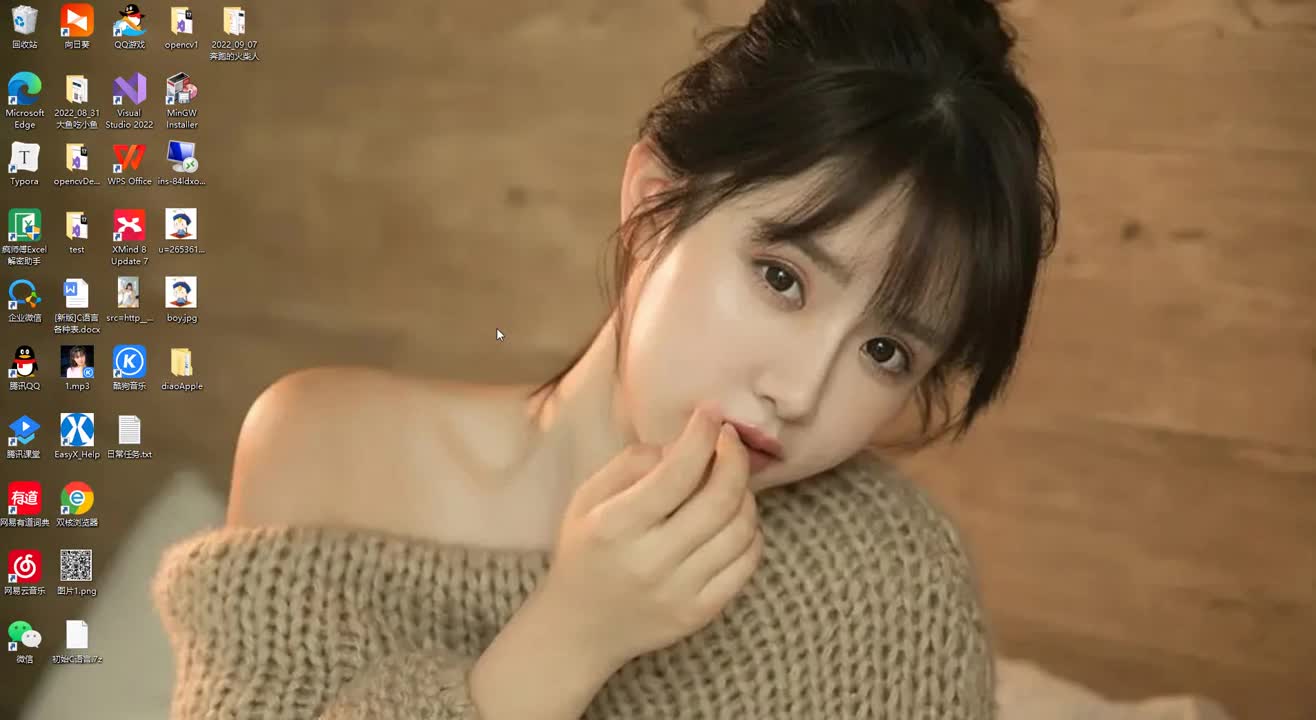
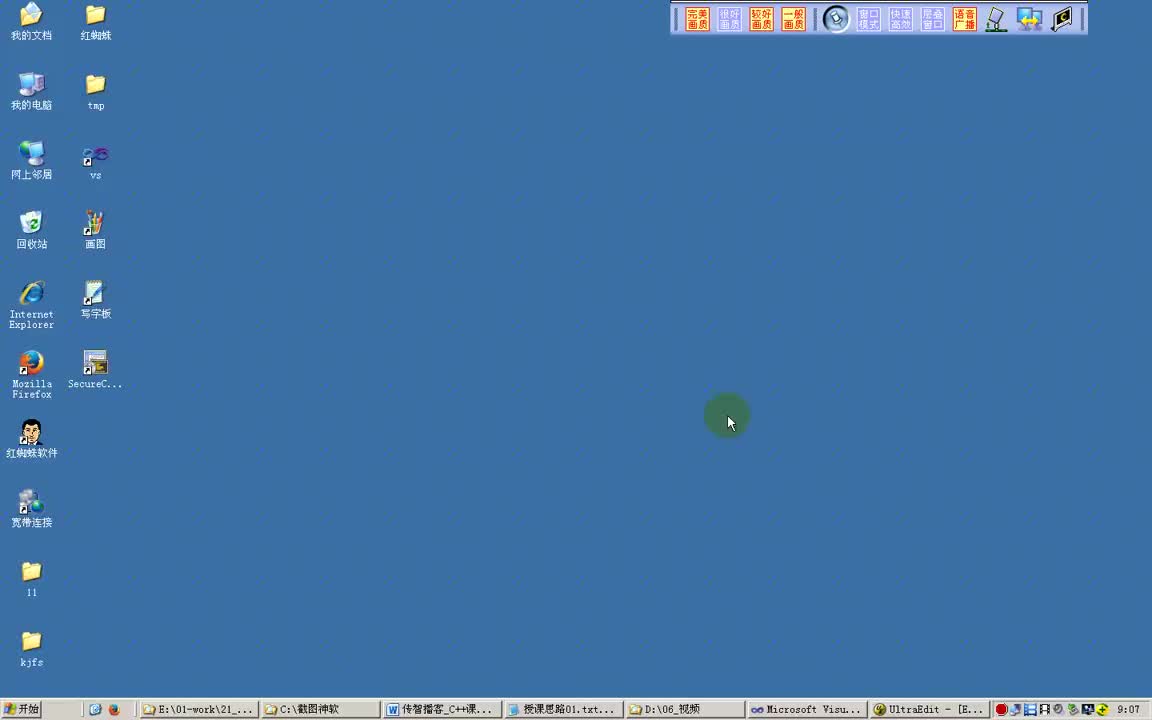
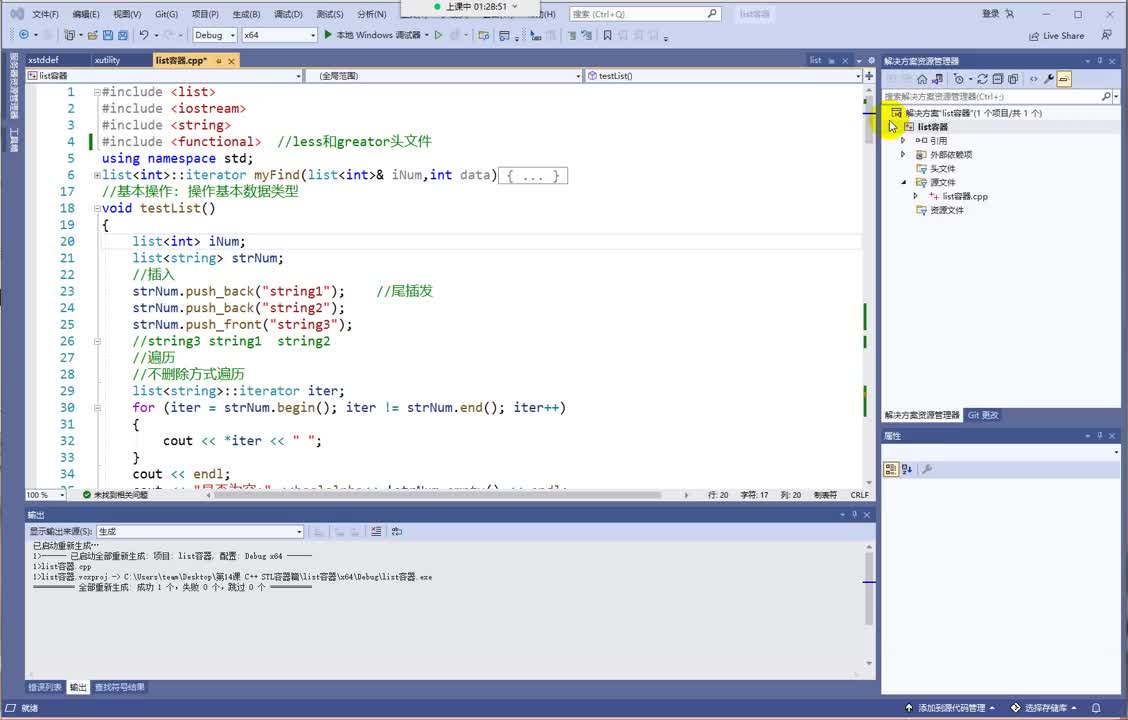
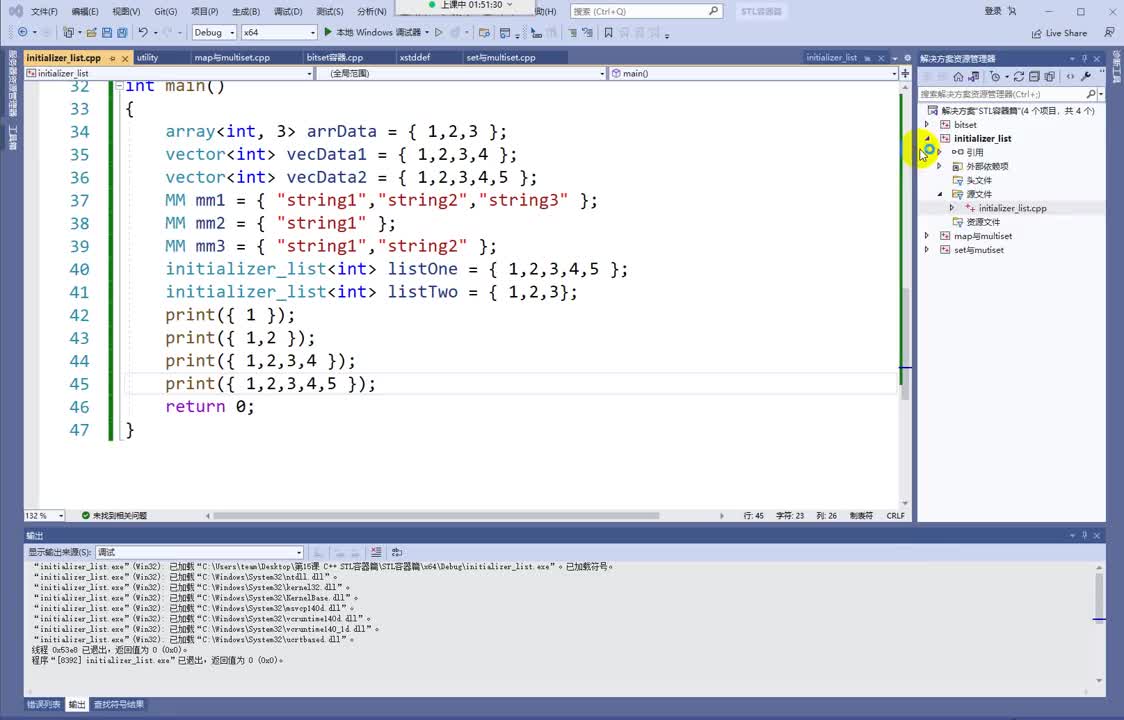
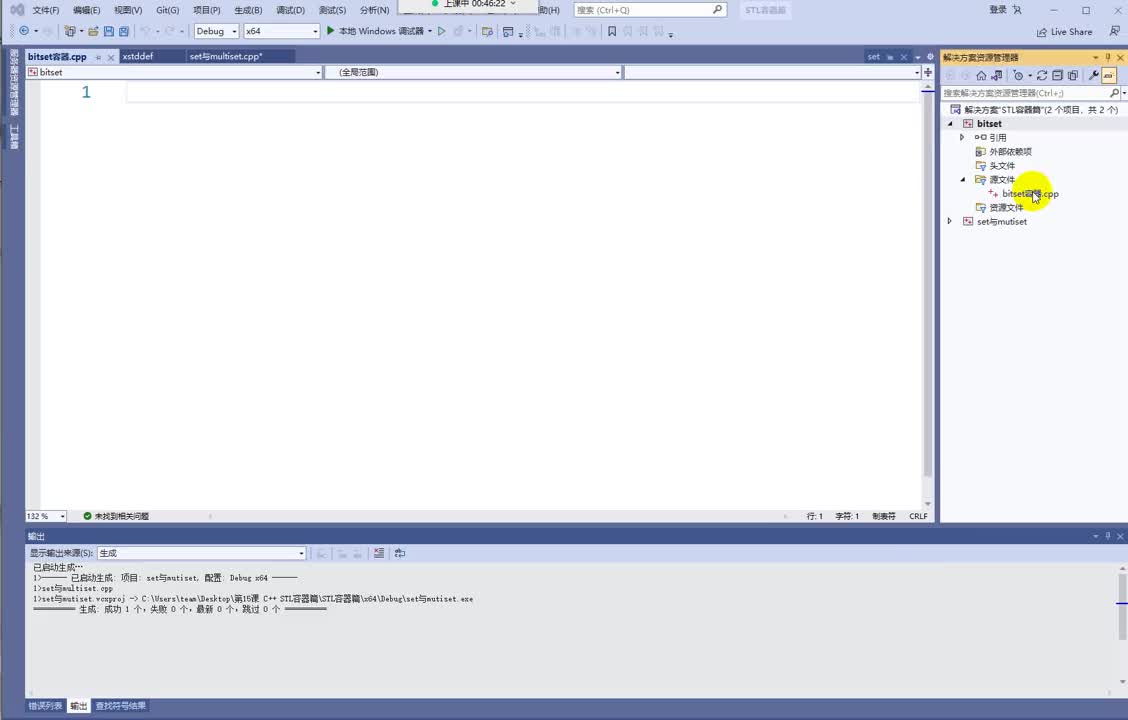
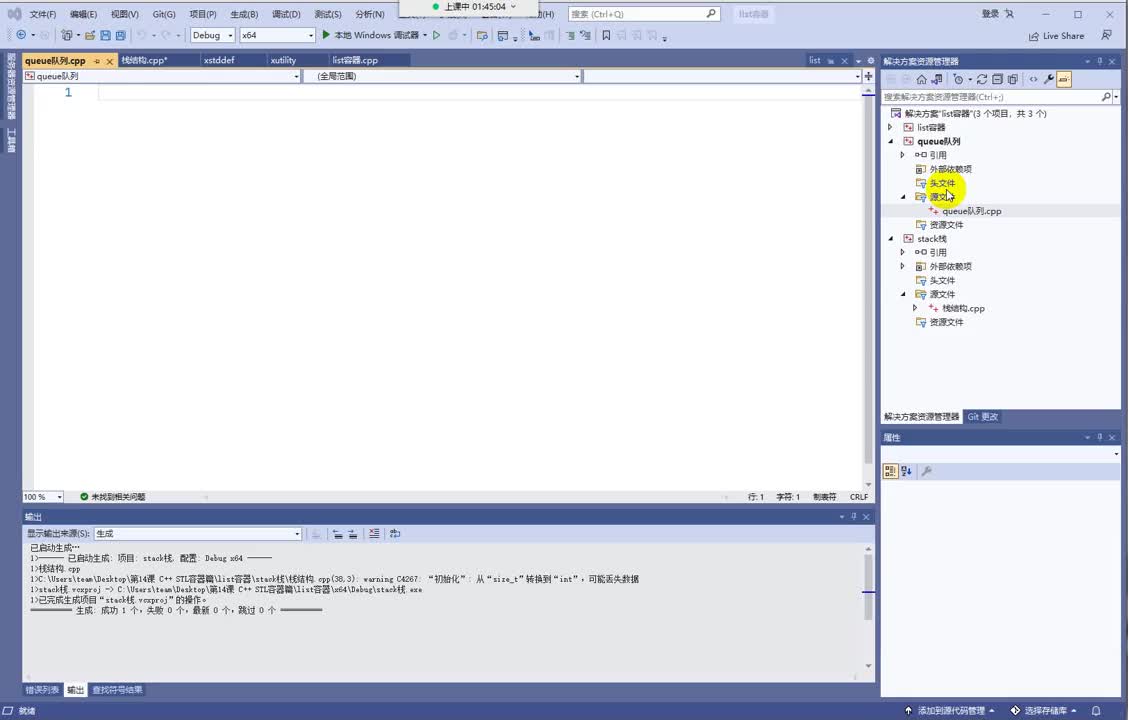
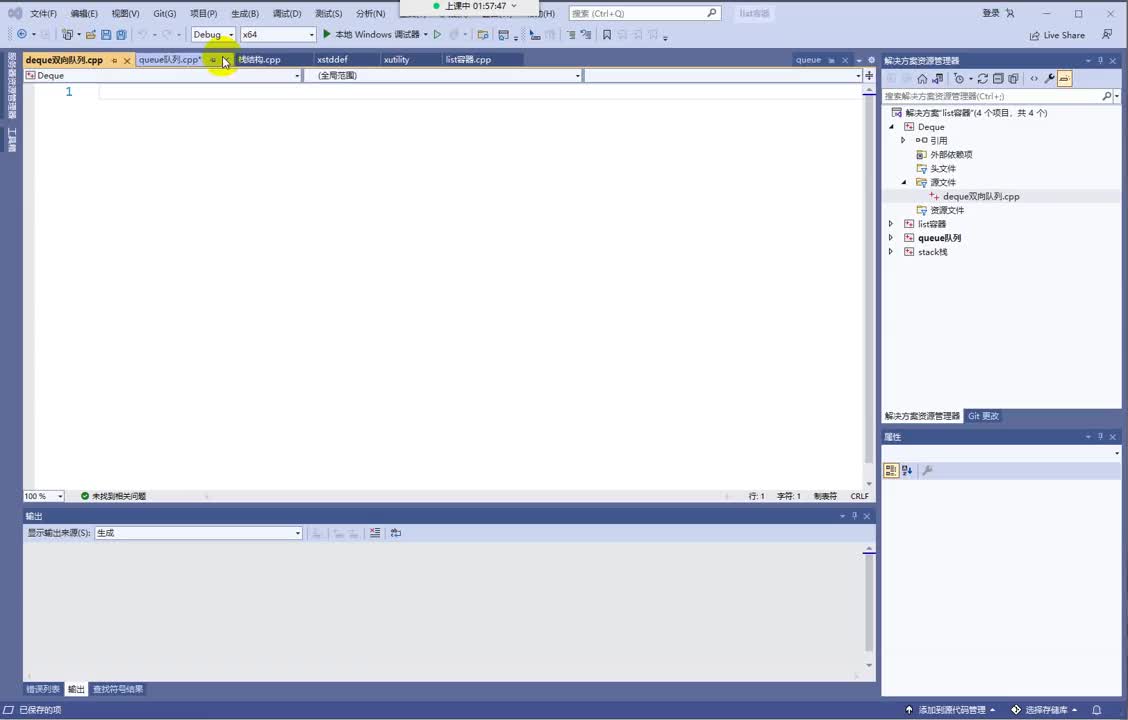
MSP430之裸奔框架C++程序源码(菜农C++裸奔大法系列之一) 转载
ccs如何使用C语言的“容器”?
C++标准库学习笔记重点
C/C++开源库及示例代码简介
在main文件中怎样去使用C++呢
详细剖析C++的的3种容器
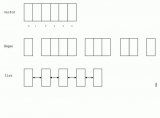
评论