一、导入elasticsearch依赖
在pom.xml里加入如下依赖
org.springframework.boot spring-boot-starter-data-elasticsearch
非常重要:检查依赖版本是否与你当前所用的版本是否一致,如果不一致,会连接失败!
基于 Spring Boot + MyBatis Plus + Vue & Element 实现的后台管理系统 + 用户小程序,支持 RBAC 动态权限、多租户、数据权限、工作流、三方登录、支付、短信、商城等功能
项目地址:https://github.com/YunaiV/ruoyi-vue-pro
视频教程:https://doc.iocoder.cn/video/
二、创建高级客户端
importorg.apache.http.HttpHost; importorg.elasticsearch.client.RestClient; importorg.elasticsearch.client.RestHighLevelClient; importorg.springframework.context.annotation.Bean; importorg.springframework.context.annotation.Configuration; @Configuration publicclassElasticSearchClientConfig{ @Bean publicRestHighLevelClientrestHighLevelClient(){ RestHighLevelClientclient=newRestHighLevelClient( RestClient.builder( newHttpHost("服务器IP",9200,"http"))); returnclient; } }
基于 Spring Cloud Alibaba + Gateway + Nacos + RocketMQ + Vue & Element 实现的后台管理系统 + 用户小程序,支持 RBAC 动态权限、多租户、数据权限、工作流、三方登录、支付、短信、商城等功能
项目地址:https://github.com/YunaiV/yudao-cloud
视频教程:https://doc.iocoder.cn/video/
三、基本用法
1.创建、判断存在、删除索引
importorg.elasticsearch.action.admin.indices.delete.DeleteIndexRequest; importorg.elasticsearch.action.support.master.AcknowledgedResponse; importorg.elasticsearch.client.RequestOptions; importorg.elasticsearch.client.RestHighLevelClient; importorg.elasticsearch.client.indices.CreateIndexRequest; importorg.elasticsearch.client.indices.CreateIndexResponse; importorg.elasticsearch.client.indices.GetIndexRequest; importorg.junit.jupiter.api.Test; importorg.springframework.beans.factory.annotation.Autowired; importorg.springframework.boot.test.context.SpringBootTest; importjava.io.IOException; @SpringBootTest classElasticsearchApplicationTests{ @Autowired privateRestHighLevelClientrestHighLevelClient; @Test voidtestCreateIndex()throwsIOException{ //1.创建索引请求 CreateIndexRequestrequest=newCreateIndexRequest("ljx666"); //2.客户端执行请求IndicesClient,执行create方法创建索引,请求后获得响应 CreateIndexResponseresponse= restHighLevelClient.indices().create(request,RequestOptions.DEFAULT); System.out.println(response); } @Test voidtestExistIndex()throwsIOException{ //1.查询索引请求 GetIndexRequestrequest=newGetIndexRequest("ljx666"); //2.执行exists方法判断是否存在 booleanexists=restHighLevelClient.indices().exists(request,RequestOptions.DEFAULT); System.out.println(exists); } @Test voidtestDeleteIndex()throwsIOException{ //1.删除索引请求 DeleteIndexRequestrequest=newDeleteIndexRequest("ljx666"); //执行delete方法删除指定索引 AcknowledgedResponsedelete=restHighLevelClient.indices().delete(request,RequestOptions.DEFAULT); System.out.println(delete.isAcknowledged()); } }
2.对文档的CRUD
创建文档:
注意:如果添加时不指定文档ID,他就会随机生成一个ID,ID唯一。
创建文档时若该ID已存在,发送创建文档请求后会更新文档中的数据。
@Test voidtestAddUser()throwsIOException{ //1.创建对象 Useruser=newUser("Go",21,newString[]{"内卷","吃饭"}); //2.创建请求 IndexRequestrequest=newIndexRequest("ljx666"); //3.设置规则PUT/ljx666/_doc/1 //设置文档id=6,设置超时=1s等,不设置会使用默认的 //同时支持链式编程如request.id("6").timeout("1s"); request.id("6"); request.timeout("1s"); //4.将数据放入请求,要将对象转化为json格式 //XContentType.JSON,告诉它传的数据是JSON类型 request.source(JSONValue.toJSONString(user),XContentType.JSON); //5.客户端发送请求,获取响应结果 IndexResponseindexResponse=restHighLevelClient.index(request,RequestOptions.DEFAULT); System.out.println(indexResponse.toString()); System.out.println(indexResponse.status()); }
获取文档中的数据:
@Test voidtestGetUser()throwsIOException{ //1.创建请求,指定索引、文档id GetRequestrequest=newGetRequest("ljx666","1"); GetResponsegetResponse=restHighLevelClient.get(request,RequestOptions.DEFAULT); System.out.println(getResponse);//获取响应结果 //getResponse.getSource()返回的是Map集合 System.out.println(getResponse.getSourceAsString());//获取响应结果source中内容,转化为字符串 }
更新文档数据:
注意:需要将User对象中的属性全部指定值,不然会被设置为空,如User只设置了名称,那么只有名称会被修改成功,其他会被修改为null。
@Test voidtestUpdateUser()throwsIOException{ //1.创建请求,指定索引、文档id UpdateRequestrequest=newUpdateRequest("ljx666","6"); Useruser=newUser("GoGo",21,newString[]{"内卷","吃饭"}); //将创建的对象放入文档中 request.doc(JSONValue.toJSONString(user),XContentType.JSON); UpdateResponseupdateResponse=restHighLevelClient.update(request,RequestOptions.DEFAULT); System.out.println(updateResponse.status());//更新成功返回OK }
删除文档:
@Test voidtestDeleteUser()throwsIOException{ //创建删除请求,指定要删除的索引与文档ID DeleteRequestrequest=newDeleteRequest("ljx666","6"); DeleteResponseupdateResponse=restHighLevelClient.delete(request,RequestOptions.DEFAULT); System.out.println(updateResponse.status());//删除成功返回OK,没有找到返回NOT_FOUND }
3.批量CRUD数据
这里只列出了批量插入数据,其他与此类似
注意:hasFailures()方法是返回是否失败,即它的值为false时说明上传成功
@Test voidtestBulkAddUser()throwsIOException{ BulkRequestbulkRequest=newBulkRequest(); //设置超时 bulkRequest.timeout("10s"); ArrayListlist=newArrayList<>(); list.add(newUser("Java",25,newString[]{"内卷"})); list.add(newUser("Go",18,newString[]{"内卷"})); list.add(newUser("C",30,newString[]{"内卷"})); list.add(newUser("C++",26,newString[]{"内卷"})); list.add(newUser("Python",20,newString[]{"内卷"})); intid=1; //批量处理请求 for(Useru:list){ //不设置id会生成随机id bulkRequest.add(newIndexRequest("ljx666") .id(""+(id++)) .source(JSONValue.toJSONString(u),XContentType.JSON)); } BulkResponsebulkResponse=restHighLevelClient.bulk(bulkRequest,RequestOptions.DEFAULT); System.out.println(bulkResponse.hasFailures());//是否执行失败,false为执行成功 }
4.查询所有、模糊查询、分页查询、排序、高亮显示
@Test voidtestSearch()throwsIOException{ SearchRequestsearchRequest=newSearchRequest("ljx666");//里面可以放多个索引 SearchSourceBuildersourceBuilder=newSearchSourceBuilder();//构造搜索条件 //此处可以使用QueryBuilders工具类中的方法 //1.查询所有 sourceBuilder.query(QueryBuilders.matchAllQuery()); //2.查询name中含有Java的 sourceBuilder.query(QueryBuilders.multiMatchQuery("java","name")); //3.分页查询 sourceBuilder.from(0).size(5); //4.按照score正序排列 //sourceBuilder.sort(SortBuilders.scoreSort().order(SortOrder.ASC)); //5.按照id倒序排列(score会失效返回NaN) //sourceBuilder.sort(SortBuilders.fieldSort("_id").order(SortOrder.DESC)); //6.给指定字段加上指定高亮样式 HighlightBuilderhighlightBuilder=newHighlightBuilder(); highlightBuilder.field("name").preTags("").postTags(""); sourceBuilder.highlighter(highlightBuilder); searchRequest.source(sourceBuilder); SearchResponsesearchResponse=restHighLevelClient.search(searchRequest,RequestOptions.DEFAULT); //获取总条数 System.out.println(searchResponse.getHits().getTotalHits().value); //输出结果数据(如果不设置返回条数,大于10条默认只返回10条) SearchHit[]hits=searchResponse.getHits().getHits(); for(SearchHithit:hits){ System.out.println("分数:"+hit.getScore()); Mapsource=hit.getSourceAsMap(); System.out.println("index->"+hit.getIndex()); System.out.println("id->"+hit.getId()); for(Map.Entry s:source.entrySet()){ System.out.println(s.getKey()+"--"+s.getValue()); } } }
四、总结
1.大致流程
创建对应的请求 --> 设置请求(添加规则,添加数据等) --> 执行对应的方法(传入请求,默认请求选项)–> 接收响应结果(执行方法返回值)–> 输出响应结果中需要的数据(source,status等)
2.注意事项
如果不指定id,会自动生成一个随机id
正常情况下,不应该这样使用new IndexRequest(“ljx777”),如果索引发生改变了,那么代码都需要修改,可以定义一个枚举类或者一个专门存放常量的类,将变量用final static等进行修饰,并指定索引值。其他地方引用该常量即可,需要修改也只需修改该类即可。
elasticsearch相关的东西,版本都必须一致,不然会报错
elasticsearch很消耗内存,建议在内存较大的服务器上运行elasticsearch,否则会因为内存不足导致elasticsearch自动killed。
审核编辑:刘清
-
RBAC
+关注
关注
0文章
43浏览量
9891 -
SpringBoot
+关注
关注
0文章
172浏览量
106
原文标题:SpringBoot+ElasticSearch 实现模糊查询,批量CRUD,排序,分页,高亮
文章出处:【微信号:芋道源码,微信公众号:芋道源码】欢迎添加关注!文章转载请注明出处。
发布评论请先 登录
相关推荐
SpringBoot整合ElasticSearch
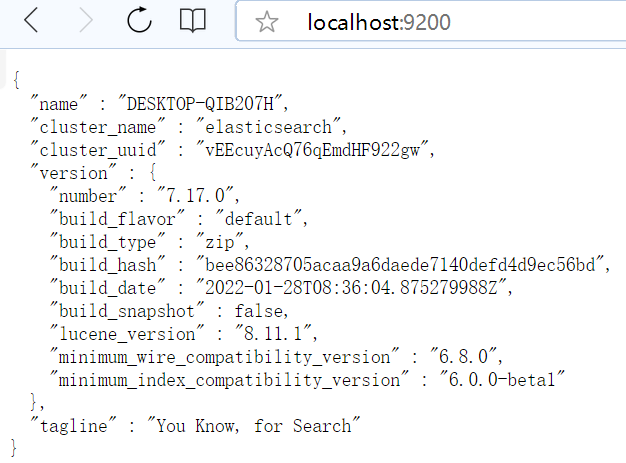
分析一下MySQL数据库与ElasticSearch的实际应用
为什么ElasticSearch复杂条件查询比MySQL好?
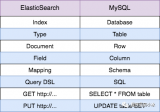
ElasticSearch是什么?应用场景是什么?
SpringBoot模板分类树查询功能介绍
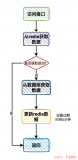
加密后的敏感字段还能进行模糊查询吗?该如何实现?
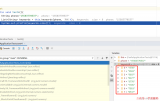
SpringBoot 连接ElasticSearch的使用方式
Redis的分页+多条件模糊查询组合实现方案
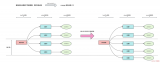
评论